How it works
Sym is the most developer-friendly way to secure your production infrastructure.
Sym is a platform for defining simple automations that turn just-in-time access policies into easy-to-operate workflows, executed in Slack. Define access flows in Terraform, customize and integrate with other systems in code, and then use our API or Slack App to request and approve/deny access. Simple!
The basic Sym workflow
Let’s say you want to create a workflow to allow specific team members to use an elevated AWS SSO Permission Set, for a specific length of time. Here’s how you’d get that started in Sym:
1. Declare your access flows in code using Terraform
In Sym’s Terraform provider, you specify which resource (Permission Set) you want to work with, what kind of access you want to grant or revoke (PowerUser), which AWS Account ID it corresponds to, etc. Your resource definition might look something like this:
resource "sym_target" "power_user" {
type = "aws_sso_permission_set"
name = "power-user"
label = "AWS Power User"
settings = {
# `type=aws_sso_permission_set` sym_targets need both an AWS Permission Set
# ARN and an AWS Account ID to make an sso account assignment
permission_set_arn = "arn:aws:sso:::permissionSet/ssoins-aaaaaaaaaaaaaaaa/ps-aaaaaaaaaaaaaaaa"
account_id = "333333333333"
}
}
Within your Terraform definitions, you also specify what information your access requesters will need to provide, like reason for access and duration:
params {
# By specifying a strategy, this Flow will now be able to manage access (escalate/de-escalate)
# to the targets specified in the `sym_strategy` resource.
strategy_id = sym_strategy.aws_iam.id
# Each prompt_field defines a custom form field for the Slack modal that
# requesters fill out to make their requests.
prompt_field {
name = "reason"
label = "Why do you need access?"
type = "string"
required = true
}
prompt_field {
name = "duration"
type = "duration"
allowed_values = ["30m", "1h"]
required = true
}
2. Use Slack to request and approve just-in-time, temporary access
Sym then takes your Terraform definitions and wraps them in a convenient Slack UI. When a team member wants to request access to a resource, they just type /sym
to begin a request.
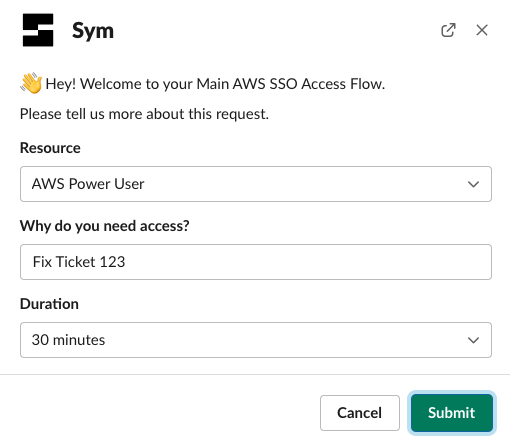
That kicks off the Terraform-defined workflow, and routes the request to the appropriate admin, who can approve or deny it.
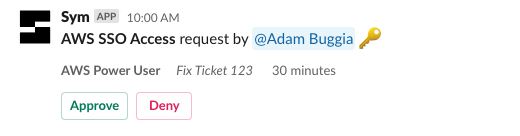
If the request is approved, Sym's workflow engine automatically revokes access at the end of the allocated duration.
Approvals API
By the way, you can also kick off workflows outside of Slack using Sym's Events API.
3. Extend with rules and integrations (the Sym SDK)
Really embracing just-in-time access usually involves more than just your infrastructure provider. Sym's SDK allows you to call out to external data sources and APIs and involve them in your workflows. Common use cases include checking a user's Okta group, or making a request to the PagerDuty API to see if a given engineer is currently on call.
from sym.sdk.annotations import hook, reducer
from sym.sdk.integrations import pagerduty, slack
from sym.sdk.templates import ApprovalTemplate
@hook
def on_request(evt):
"""
If this is a fast-tracked request, then auto-approve the workflow.
"""
fvars = evt.flow.vars
if is_fast_tracked(evt):
target = evt.payload.fields["target"]
message = f"{evt.user.email} was fast tracked {target.label} AWS access."
return ApprovalTemplate.approve(reason=message)
def is_fast_tracked(evt):
"""
Determine if this request should be fast tracked or go through
normal approval channels
"""
return pagerduty.is_on_call(evt.user)
Sym's goal is to help you codify as many of these kinds of manual rules (e.g. checking if someone is on call) as possible to make access more secure and predictable.
Audit logging and reporting
Every request, approval, and denial is logged to make reporting easier for you. You can define a log destination like Kinesis, Datadog, etc. and Sym will pipe audit logs straight there. Logs contain rich metadata about every workflow action, and complement Sym's policies-as-code by providing easy evidence collection for certifications like SOC II.
Updated 5 months ago