Jira SDK Integration
Sym provides SDK methods that allow you to interact with Jira tickets as part of codifying your access request process.
The Sym SDK provides convenience methods for interacting with Jira in your impl.py
.
Install the Sym SDK app for Jira Connect
Install the official Sym SDK app for Jira Connect through the Atlassian Marketplace by clicking "Get it now."
After the Jira Connect app has installed, click "Get Started" in the pop-up and follow the instructions provided, or click "Get Started" from the Atlassian Marketplace page.
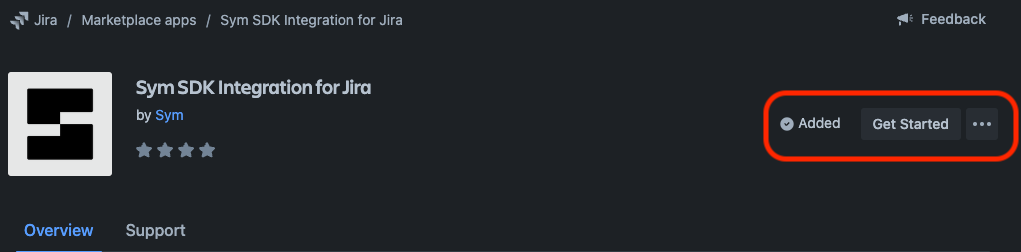
Using Jira in your Flows
For more information on the supported methods in the jira
module, please visit the Sym SDK Jira Docs.
Example Implementations
Provide a list of Jira Tickets in a prefetch prompt field
from sym.sdk.annotations import prefetch
from sym.sdk.integrations import jira
from sym.sdk.forms import FieldOption
@prefetch(field_name="jira_ticket")
def get_jira_tickets(event):
issues = jira.search_issues(jql="project = SP")
return [
FieldOption(value=issue["key"], label=issue["key"])
for issue in issues
]
resource "sym_flow" "jira" {
name = "jira"
label = "Approve Jira Ticket"
implementation = file("./impl.py")
environment_id = sym_environment.this.id
params {
prompt_field {
name = "jira_ticket"
label = "Jira Ticket"
type = "string"
required = true
prefetch = true
}
prompt_field {
name = "reason"
label = "What do you need approved?"
type = "string"
required = true
}
}
}
Add a comment to Jira Tickets which are past their due date
from sym.sdk.integrations import jira
def add_followup_comment_if_duedate_has_passed():
issues = jira.search_issues(jql="project = SP and status != 'Done' and duedate < now()")
for issue in issues:
jira.add_comment_to_issue(issue_id_or_key=issue["id"], comment="Hey, what's the update on this?")
Add a comment to Jira Ticket after selecting it in a request
from sym.sdk.integrations import jira
@hook
def after_request(event):
jira_ticket_id = event.payload.fields["jira_ticket"]
jira.add_comment_to_issue(
issue_id_or_key=jira_ticket_id,
comment="A request has been made related to this ticket"
)
Create a Jira Ticket when a request is made
from sym.sdk.integrations import jira
@hook
def after_request(event):
created_issue = jira.create_issue(project_key="SYM", issue_type_name="Task", title=f"Access Request for {event.payload.fields["target"].label} by {event.user.email}", labels=["sym_request"])
print(f"The Jira ticket {created_issue} has been created!")
Updated 5 months ago